策略模式,是算法族的集合,将算法抽象出接口,并根据接口实现各种算法。在使用算法的具体类中设置相应的算法,为什么有策略模式的诞生,建议大家看下Headfirst,里边的介绍是相当的详细,看完后保证有茅塞顿开的感觉。
UML图:
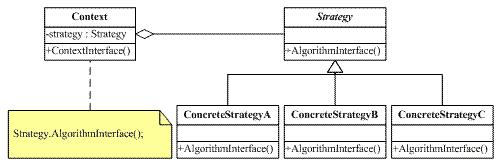
策略模式中讲究的是组合,算法族的建立。
本文的算法族是英雄的行动IAction接口。Dota中的英雄有走着的,有飞行的,有爬行的。分别写行走类,飞行类,爬行类继承IAction接口。
IAction可以在构造函数中设置,也可以在客户端调用时动态设置,有着很灵活的状态。如果加入新行动类是并不会影响其他行动类。
IAction接口如下:
public interface IAction
{
void Action();
}
具体各个行动类如下:
UML图:
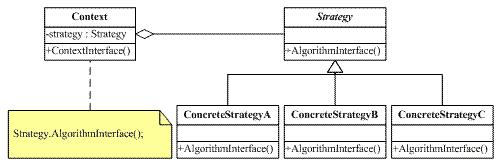
策略模式中讲究的是组合,算法族的建立。
本文的算法族是英雄的行动IAction接口。Dota中的英雄有走着的,有飞行的,有爬行的。分别写行走类,飞行类,爬行类继承IAction接口。
IAction可以在构造函数中设置,也可以在客户端调用时动态设置,有着很灵活的状态。如果加入新行动类是并不会影响其他行动类。
IAction接口如下:
public interface IAction
{
void Action();
}
具体各个行动类如下:
/// <summary>
/// 行走
/// </summary>
public class Walk : IAction
{
#region IAction 成员
{
LandpyForm.Form.OutputResult("I walk.");
}
}
/// 飞行
/// </summary>
public class Fly : IAction
{
#region IAction 成员
{
LandpyForm.Form.OutputResult("I Fly.");
}
}
/// 爬行
/// </summary>
public class Crawl : IAction
{
#region IAction 成员
{
LandpyForm.Form.OutputResult("I Crawl.");
}
}
/// 行走
/// </summary>
public class Walk : IAction
{
#region IAction 成员
public void Action()
{
LandpyForm.Form.OutputResult("I walk.");
}
#endregion
}
/// <summary>
/// 飞行
/// </summary>
public class Fly : IAction
{
#region IAction 成员
public void Action()
{
LandpyForm.Form.OutputResult("I Fly.");
}
#endregion
}
/// <summary>
/// 爬行
/// </summary>
public class Crawl : IAction
{
#region IAction 成员
public void Action()
{
LandpyForm.Form.OutputResult("I Crawl.");
}
#endregion
}
测试代码:
DotaPatternLibrary.Strategy.Hero hero = new DotaPatternLibrary.Strategy.Terrorblade();
hero.DoAction();
hero = new DotaPatternLibrary.Strategy.Syllabear();
hero.DoAction();
LandpyForm.Form.OutputResult("变身");
hero.SetAction(new DotaPatternLibrary.Strategy.Crawl());
hero.DoAction();
hero = new DotaPatternLibrary.Strategy.Puck();
hero.DoAction();
完整代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text; using DotaCommon; namespace DotaPatternLibrary.Strategy
{
public interface IAction
{
void Action();
}
/// 行走
/// </summary>
public class Walk : IAction
{
#region IAction 成员
{
LandpyForm.Form.OutputResult("I walk.");
}
}
/// 飞行
/// </summary>
public class Fly : IAction
{
#region IAction 成员
{
LandpyForm.Form.OutputResult("I Fly.");
}
}
/// 爬行
/// </summary>
public class Crawl : IAction
{
#region IAction 成员
{
LandpyForm.Form.OutputResult("I Crawl.");
}
}
/// 英雄
/// </summary>
public abstract class Hero
{
protected IAction action;
public void DoAction()
{
action.Action();
}
{
this.action = action;
}
}
/// 灵魂守卫
/// </summary>
public class Terrorblade : Hero
{
public Terrorblade()
{
LandpyForm.Form.OutputResult("创建灵魂守卫");
action = new Walk();
}
}
/// 德鲁伊
/// </summary>
public class Syllabear : Hero
{
public Syllabear()
{
LandpyForm.Form.OutputResult("创建德鲁伊");
action = new Walk();
}
}
/// 精灵龙
/// </summary>
public class Puck : Hero
{
public Puck()
{
LandpyForm.Form.OutputResult("创建精灵龙");
action = new Fly();
}
}
}
using System.Collections.Generic;
using System.Linq;
using System.Text; using DotaCommon; namespace DotaPatternLibrary.Strategy
{
public interface IAction
{
void Action();
}
/// <summary>
/// 行走
/// </summary>
public class Walk : IAction
{
#region IAction 成员
public void Action()
{
LandpyForm.Form.OutputResult("I walk.");
}
#endregion
}
/// <summary>
/// 飞行
/// </summary>
public class Fly : IAction
{
#region IAction 成员
public void Action()
{
LandpyForm.Form.OutputResult("I Fly.");
}
#endregion
}
/// <summary>
/// 爬行
/// </summary>
public class Crawl : IAction
{
#region IAction 成员
public void Action()
{
LandpyForm.Form.OutputResult("I Crawl.");
}
#endregion
}
/// <summary>
/// 英雄
/// </summary>
public abstract class Hero
{
protected IAction action;
public void DoAction()
{
action.Action();
}
public void SetAction(IAction action)
{
this.action = action;
}
}
/// <summary>
/// 灵魂守卫
/// </summary>
public class Terrorblade : Hero
{
public Terrorblade()
{
LandpyForm.Form.OutputResult("创建灵魂守卫");
action = new Walk();
}
}
/// <summary>
/// 德鲁伊
/// </summary>
public class Syllabear : Hero
{
public Syllabear()
{
LandpyForm.Form.OutputResult("创建德鲁伊");
action = new Walk();
}
}
/// <summary>
/// 精灵龙
/// </summary>
public class Puck : Hero
{
public Puck()
{
LandpyForm.Form.OutputResult("创建精灵龙");
action = new Fly();
}
}
}