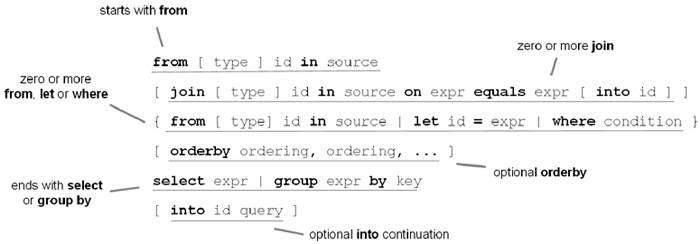
(来自Linq in action)
举个例子实现:
查询以a开头的字符串,按降序输出。
C#代码:
var result
= from word in listwhere word.StartsWith("a")
orderby word descending
select word;
result.ToList().ForEach(str
=> Console.WriteLine(str)); // 输出:// async
// apple
// amazon
// adobe
// acer
等价的面向对象语法为:
.OrderByDescending(str => { return str; });
这里 Where 和 OrderByDescending都是IEnumerable<T>的扩展方法,因为数组类型实现了该接口,所以可以直接调用这些这些扩展方法。
这些扩展方法的实现是在Enumerable类型中实现的,因此,更直接的是调用Enumerable类型的静态方法方法:
Enumerable.OrderByDescending(
Enumerable.Where(
list, str => str.StartsWith("a")), str => { return str; }
);
从这些逆向演化可以看出,查询语法就是一种"语法糖"。前面提到过,编译后运行时版本还是2.0,从这可以看出一些端倪。如果想继续深入了解该实现可以用ILDASM查看IL源代码和反射类库源代码。
result类型为IEnumerable<TElement>或派生自IEnumerable<TElement>接口的类型。
与 Linq 相关的程序集
System.Linq 命名空间在System.Core.dll中,接口类型及扩展方法的定义在该命名空间中,如:
Enumerable
IGrouping<TKey, TElement>
ILookup<TKey, TElement>
IQueryable<T>
IOrderedQueryable<T>
Expressions
...
System.Data.DataSetExtensions.dll 包含 Linq to DataSet 的相关类型的定义。
System.Data.Linq.dll 包含 Linq to SQL 的相关类型的定义。
System.Xml.Linq.dll 包含 Linq to XML 的相关类型的定义。
全程数据
为了下面介绍方便,构造以下,
数据结构图如下:
数据定义如下:
public class Student
{
public int StudentID { get; set; }
public string Name { get; set; }
override public string ToString()
{
return string.Format("Student ID:{0},Student Name:{1}", StudentID, Name);
}
}
public class Course
{
public int CourseID { get; set; }
public string CourseName { get; set; }
override public string ToString()
{
return string.Format("Course ID:{0},Course Name:{1}", CourseID, CourseName);
}
}
public class Score
{
public int StudentID { get; set; }
public int CourseID { get; set; }
public double Value { get; set; }
override public string ToString()
{
return string.Format("Student ID:{0},Course ID:{1},Score:{2}", StudentID, CourseID, Value);
}
}
public class DataSource
{
public static List<Student> Students { get; private set; }
public static List<Student> Students2 { get; private set; }
public static List<Score> Scores { get; private set; }
public static List<Course> Courses { get; private set; }
static DataSource()
{
Students = new List<Student>
{
new Student{ StudentID=1, Name="Andy" },
new Student{ StudentID=2, Name="Bill" },
new Student{ StudentID=3, Name="Cindy" },
new Student{ StudentID=4, Name="Dark" }
};
Students2 = new List<Student>
{
new Student{ StudentID=5, Name="Erik" },
new Student{ StudentID=6, Name="Frank" }
};
Courses = new List<Course>
{
new Course { CourseID = 1, CourseName = "C Language" },
new Course { CourseID = 2, CourseName = "Biophysics" },
new Course { CourseID = 3, CourseName = "Fundamentals of Compiling" },
new Course { CourseID = 4, CourseName = "Finance" },
new Course { CourseID = 5, CourseName = "College English" }
};
Scores = new List<Score>
{
new Score { StudentID = 1, CourseID = 1, Value = 78 },
new Score { StudentID = 1, CourseID = 2, Value = 60 },
new Score { StudentID = 1, CourseID = 3, Value = 92 },
new Score { StudentID = 1, CourseID = 4, Value = 85 },
new Score { StudentID = 1, CourseID = 5, Value = 55.5 },
new Score { StudentID = 2, CourseID = 1, Value = 59 },
new Score { StudentID = 2, CourseID = 2, Value = 78 },
new Score { StudentID = 2, CourseID = 3, Value = 86 },
new Score { StudentID = 3, CourseID = 2, Value = 60 },
new Score { StudentID = 4, CourseID = 4, Value = 96 },
new Score { StudentID = 5, CourseID = 5, Value = 78 }
};
}
}
后续知识点将使用这里定义的数据进行解析。