最近不少朋友对我以前发的一些例子中的流程设计器有一些疑问,以后我会专门写一个流程设计器的例子,这里先写几个开发流程设计器时要用到的小知识点
1.为自定义的Activity添加图标
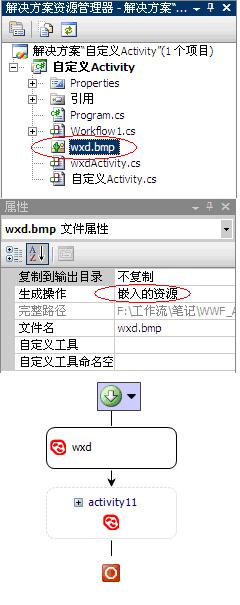
为自定义Activity设置成员属性
[System.Drawing.ToolboxBitmap(typeof(wxdActivity), "wxd.bmp")]
public class wxdActivity : System.Workflow.ComponentModel.Activity
2.为Activity设置外观
//设计器样式类
[ActivityDesignerThemeAttribute(typeof(wxdTheme))]
public class wxdActivityDesigner : ActivityDesigner

{
}

//主题类
public class wxdTheme : ActivityDesignerTheme

{
public wxdTheme(WorkflowTheme theme)
: base(theme)

{
this.BorderColor = Color.Red; //边框色
this.BorderStyle = DashStyle.Dash ; //边框线条
this.BackColorStart = Color.White; //渐变开始色
this.BackColorEnd = Color.Blue; //渐变结束色
this.BackgroundStyle = LinearGradientMode.ForwardDiagonal;//渐变方向
}
}
为自定义Activity设置成员属性
[Designer(typeof(wxdActivityDesigner), typeof(IDesigner))]
public class wxdActivity : System.Workflow.ComponentModel.Activity
如果在继承了SequenceActivity的Activity使用了上面方式定义的主题,可以使用其内部Activity结构不显示,有时不想在流程设计器中对用户暴露太多信息可以用这个方法
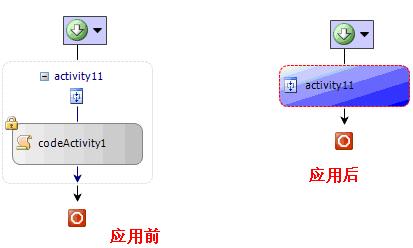
3.为Activity添加右键菜单与数据绑定窗体
有时使用属性栏对Activity进行设置,对用户来说不是很方便,比如有些属性值是用户名,设备名等要从数据库中动态加载的数据,这时为最好提供一个数据绑定窗体向导
using System.Workflow.ComponentModel;
using System.ComponentModel;
using System.Workflow.ComponentModel.Design;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.ComponentModel.Design;
using System;
using System.Collections.Generic;
namespace wxwinter


{
//自定义Activity
[Designer(typeof(wxdActivityDesigner), typeof(IDesigner))]
public class wxdActivity : System.Workflow.ComponentModel.Activity

{
public static DependencyProperty wxdValueProperty =

DependencyProperty.Register("wxdValue", typeof(string), typeof(wxdActivity));

[Browsable(true)]
[DesignerSerializationVisibility

(DesignerSerializationVisibility.Visible)]
public string wxdValue

{
get

{
return ((string)(base.GetValue(wxdActivity.wxdValueProperty)));
}
set

{
base.SetValue(wxdActivity.wxdValueProperty, value);
}
}

}


//自定义设计器
public class wxdActivityDesigner : ActivityDesigner

{
protected override

System.Collections.ObjectModel.ReadOnlyCollection<DesignerAction> DesignerActions

{
get

{
List<DesignerAction> list = new List<DesignerAction>();
foreach (DesignerAction temp in base.DesignerActions)

{
list.Add(new DesignerAction(this, temp.ActionId, temp.Text));
}
return list.AsReadOnly();
}
}
protected override ActivityDesignerVerbCollection Verbs

{
get

{
ActivityDesignerVerbCollection NewVerbs = new

ActivityDesignerVerbCollection();
NewVerbs.AddRange(base.Verbs);

ActivityDesignerVerb menu = new ActivityDesignerVerb(this,

DesignerVerbGroup.View, "设置wxdValue", new EventHandler(menu_click));
NewVerbs.Add(menu);

return NewVerbs;
}
}

//当添加的菜单单击时要执行的操作
private void menu_click(object sender, EventArgs e)

{
using (wxdSetForm wf = new wxdSetForm())

{
string v = (string)this.Activity.GetValue

(wxdActivity.wxdValueProperty);

wf.Value = v;
wf.ShowDialog();
this.Activity.SetValue(wxdActivity.wxdValueProperty,wf.Value);
}
}
}

//自定义数据设置窗体
public class wxdSetForm : System.Windows.Forms.Form

{
public string Value;
System.Windows.Forms.TextBox tx = new System.Windows.Forms.TextBox();
System.Windows.Forms.Button bt = new System.Windows.Forms.Button();
public wxdSetForm()

{
bt.Top=30;
this.Controls.Add(tx);
this.Controls.Add(bt);
bt.Click += new EventHandler(bt_Click);
bt.Text = "确定";
this.Load += new EventHandler(wxdSetForm_Load);
}

void wxdSetForm_Load(object sender, EventArgs e)

{
this.tx.Text = Value;
}

void bt_Click(object sender, EventArgs e)

{
this.Value = this.tx.Text;
this.Hide();
}
}
}
以上生成的Activity可以在VS中使用,也可以在我以前的流程设计器例子中使用
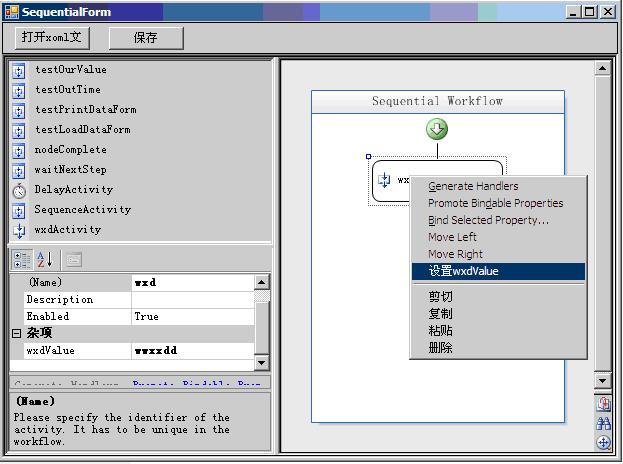
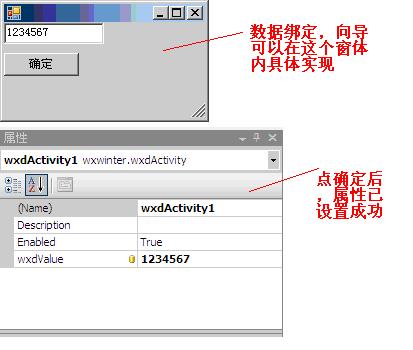
4.为Activity添加属性验证器
using System.Workflow.ComponentModel;
using System.ComponentModel;
using System.Workflow.ComponentModel.Design;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.ComponentModel.Design;
using System;
using System.Collections.Generic;
using System.Workflow.ComponentModel.Compiler;
namespace wxwinter


{
//自定义Activity
[ActivityValidator(typeof(wxdActivityValidator))]
public class wxdActivity : System.Workflow.ComponentModel.Activity

{
public static DependencyProperty wxdValueProperty =

DependencyProperty.Register("wxdValue", typeof(string), typeof(wxdActivity));

[Browsable(true)]
[DesignerSerializationVisibility

(DesignerSerializationVisibility.Visible)]
public string wxdValue

{
get

{
return ((string)(base.GetValue(wxdActivity.wxdValueProperty)));
}
set

{
base.SetValue(wxdActivity.wxdValueProperty, value);
}
}

}
//验证器类
public class wxdActivityValidator :

System.Workflow.ComponentModel.Compiler.ActivityValidator

{
public override ValidationErrorCollection ValidateProperties

(ValidationManager manager, object obj)

{
ValidationErrorCollection Errors = base.ValidateProperties(manager,

obj);

if (obj != null)

{
this.wxdValueValidator(Errors, (wxdActivity)obj);
}
return Errors;
}

private void wxdValueValidator(ValidationErrorCollection Errors,

wxdActivity obj)

{
if (obj.wxdValue=="")

{
Errors.Add(ValidationError.GetNotSetValidationError

(wxdActivity.wxdValueProperty.Name));
}
}

}

}
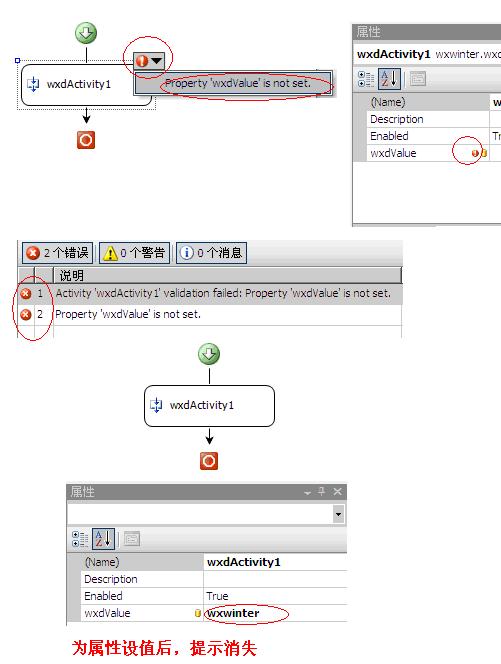
5.运行时动态将字符串编译为C#可执行代码
(与WF无关,这是C#的基础知识,在作流程设计器时有时会用到)